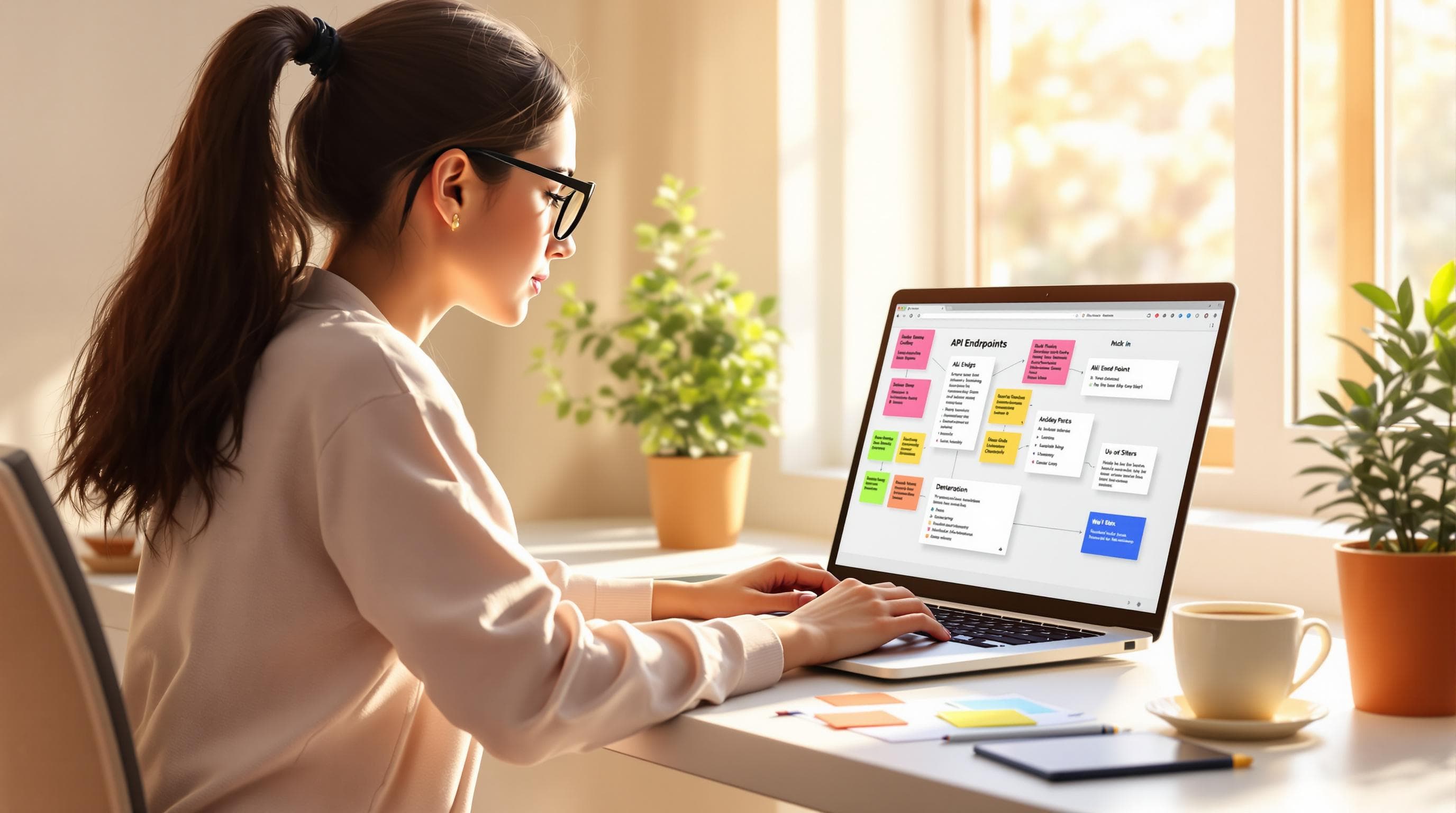
How to Mock API Endpoints for Faster Development
API mocking saves time and simplifies development. It lets you simulate APIs without waiting for the backend, enabling teams to work independently, test edge cases, and reduce costs. Here's how it helps:
- Speeds Up Development: Frontend and backend teams can work in parallel using mock APIs.
- Tests Edge Cases: Simulate errors like timeouts, server failures, and authentication issues.
- Cuts Costs: Avoid infrastructure expenses and third-party service fees during development.
- Improves Testing: Create a stable environment to test responses without relying on live systems.
Tools like ReqRes make API mocking easy. With features like traffic monitoring, endpoint simulation, and local file mapping, ReqRes helps developers debug and test efficiently. It even supports CORS and custom delays for real-world scenarios.
To get started, define your mock endpoints, align them with production APIs, and document them clearly. Incorporate mocks into your CI/CD pipeline to ensure consistent testing and smooth workflows.
Benefits of API Mocking
API mocking helps streamline workflows and improve productivity, making it easier for teams to collaborate and deliver projects efficiently.
Speed Up Team Workflows
With API mocking, teams can work on different parts of a project simultaneously. For example, frontend developers can start building features using mock endpoints while backend developers focus on implementing the actual APIs. This parallel approach keeps projects moving smoothly and avoids delays in complex workflows.
Test Edge Cases and Errors
Mock APIs allow developers to test scenarios that are hard to replicate in live systems. These scenarios include:
- Authentication failures
- Network timeouts
- Server errors
- Rate limiting responses
- Data validation issues
By simulating these situations, teams can identify and fix potential problems early, ensuring the application runs smoothly even under unexpected conditions.
Reduce Development Costs
Using API mocking can significantly lower development expenses.
"One of the primary reasons companies adopt API mocking is the cost savings it offers. By mocking services, you avoid the expenses associated with setting up or utilizing actual services during the early stages of development."
Cost Area | Savings Impact |
---|---|
Infrastructure | No need for dedicated testing environments |
Third-party Services | Avoids fees for external services during development |
Development Time | Speeds up workflows and reduces delays |
Error Prevention | Fixes problems early when they’re less costly |
API Mocking with ReqRes
ReqRes simplifies API mocking with its macOS-native application, handling nearly 500 million requests each month for consistent performance.
Key Features of ReqRes
ReqRes provides powerful HTTP(S) debugging and mocking tools tailored for macOS users. Here’s what it offers:
Feature | Description | Benefits |
---|---|---|
Traffic Monitoring | Intercept HTTP(S) requests and responses in real-time | Gain instant insights into API behavior |
Endpoint Mocking | Supports GET, POST, PUT, and DELETE methods | Simulate a fully functional REST API |
Local File Mapping | Use local files as response content with Map Local Tool | Test quickly with local data |
Its CORS-enabled setup allows requests from any domain, making it perfect for cross-origin development. You can even simulate loading delays by adding ?delay=<a number of seconds>
to any endpoint URL.
Getting Started with ReqRes
Starting with ReqRes is straightforward. Follow these steps to set up and mock APIs effectively:
-
Set Up Endpoints
Use the base URL, for instancehttps://mysite.com/
to access pre-configured mock endpoints. All endpoints support theapplication/json
content type, mirroring production API behavior. -
Simulate Authentication
Create login flows by returning tokens, 403 errors, or custom status codes. -
Modify Response Data
Customize endpoints by mapping responses to local files and defining specific HTTP status codes.
ReqRes provides reliable access for developers. Its macOS-native design ensures smooth debugging while maintaining excellent performance.
Next, explore how to set up endpoints and monitor your API traffic.
Creating Mocked Endpoints
Add Error Responses
You can simulate error responses by specifying HTTP status codes, error messages, and delays. Here are some examples:
-
Authentication errors: Return a
401
status code with a message like "Authentication failed." -
Resource not found: Use a
404
status code with details about the missing resource. -
Server errors: Return a
500
-level status code to mimic backend issues.
To test timeout handling, you can introduce response delays. For instance, the following configuration delays the response by 5000ms:
{
"endpoint": "/api/users/timeout",
"method": "GET",
"delay": 5000,
"response": {
"error": "Request timeout",
"status": 408
}
}
These setups allow you to test how your API handles failures and slow responses, ensuring better preparation for real-world conditions.
API Mocking Guidelines
After creating your endpoints, follow these tips to ensure your mocks stay accurate and development runs smoothly.
Stay Aligned with Production APIs
Mocks should mirror your production APIs. Start by defining API contracts early in the design process to validate assumptions and test behaviors. This approach helps catch integration problems before they escalate.
Here’s how to keep mocks aligned with production APIs:
- Version Control: Store mock definitions alongside your API specifications.
- Contract Testing: Regularly check mocks against your API specs.
- Routine Updates: Adjust mocks whenever production APIs are updated.
For generating dynamic data, use OpenAPI definitions to create realistic responses automatically. This ensures your mocked endpoints consistently reflect production schemas and formats.
Staying aligned with production APIs simplifies documentation and ensures effective testing.
Document Your APIs
Use OpenAPI specs to document your mocked APIs and improve team collaboration.
When documenting:
- Define Specifications: Clearly outline endpoint behaviors, data formats, and authentication requirements.
- Add Examples: Include sample requests and responses for common scenarios.
- Highlight Limitations: Note any mock-specific constraints or behaviors.
Host your mock servers at the same path level as your production APIs. This minimizes the need for code changes when transitioning to production.
Use Mocks in Testing
Incorporate mocks into your CI/CD pipeline for consistent API validation. Create detailed mocks to simulate various scenarios, such as:
Scenario Type | Example Cases | Testing Focus |
---|---|---|
Performance | High load, Timeouts | Response times, System stability |
Error Handling | Network failures, Invalid data | Error recovery, User feedback |
Security | Auth failures, Access control | Security protocols, Data protection |
Adding these mocks to your CI/CD process ensures continuous testing throughout the development cycle. This helps detect issues early and keeps your API functioning as expected while providing a smooth user experience.
Wrapping Up
API mocking plays a crucial role in today’s development workflows. Using proper mocking methods, teams can cut costs, speed up development, and reduce risks while maintaining high-quality output.
Tools like ReqRes make this process even smoother. With its native macOS design and simple one-click setup, it streamlines workflows. Plus, features like real-time monitoring and the ability to mimic different network conditions allow for thorough testing across various scenarios - perfect for teams working on tight development timelines.
By combining the right tools with smart practices, teams can keep their development cycles efficient without sacrificing thorough testing. Here are some tips to get the most out of API mocking:
- Ensure mock APIs stay aligned with production APIs.
- Provide clear and detailed documentation for all endpoints.
- Incorporate mocks into your CI/CD pipelines.
- Regularly test edge cases and error responses.