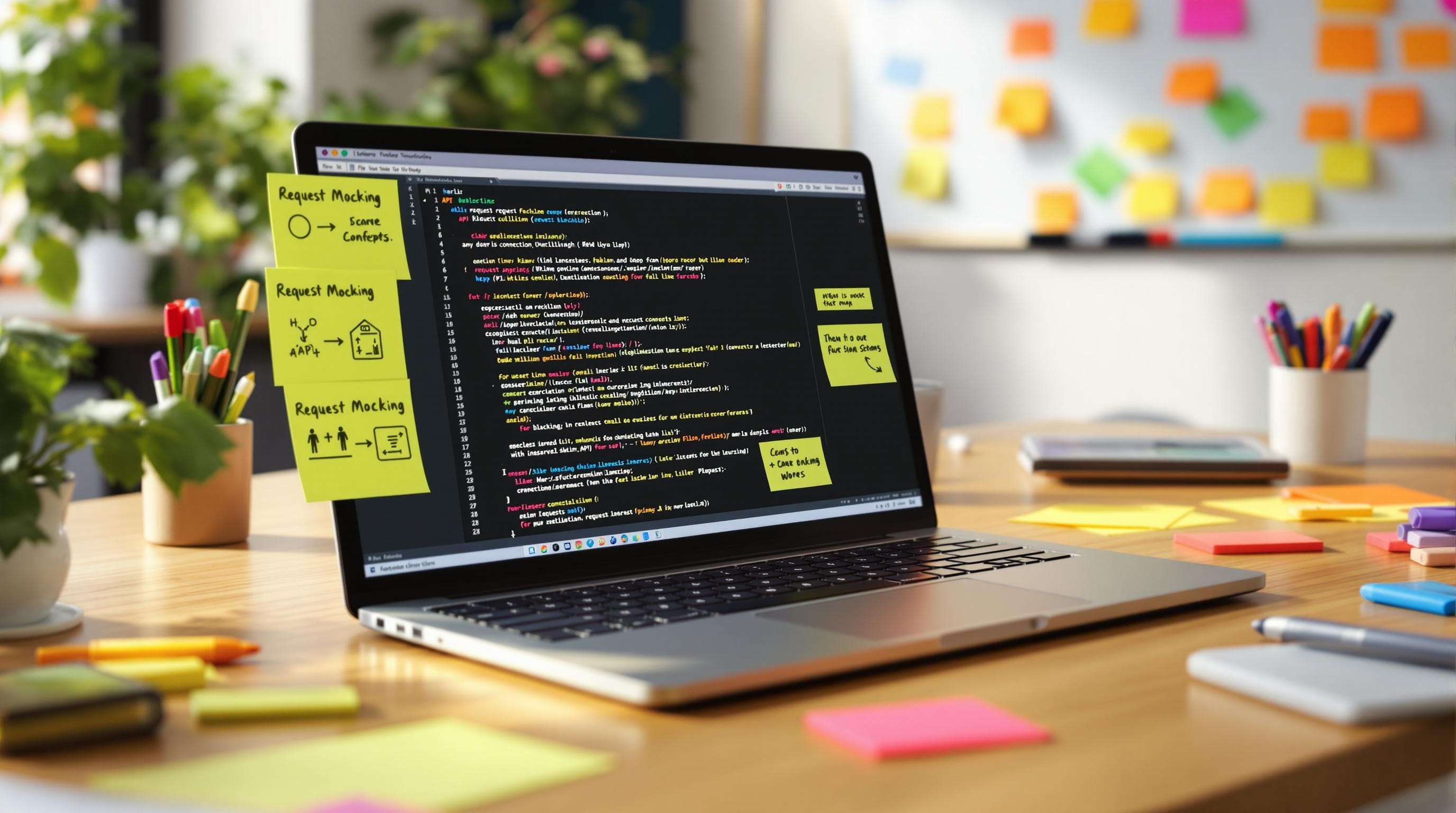
5 Ways to Speed Up API Development with Request Mocking
Want to develop APIs faster without waiting on backend teams? Request mocking is the answer. It lets you simulate API behavior, so you can test, debug, and build features even when backend services are unavailable or incomplete. Here’s a quick breakdown of how it helps:
- Work in Parallel: Frontend and backend teams can progress simultaneously.
- Test Edge Cases: Simulate errors (e.g., 401, 500) and network issues.
- Save Costs: Avoid live API fees during testing.
- Boost Efficiency: Use mock servers, dynamic rules, or local files for faster workflows.
- Automate Testing: Integrate mocks into CI/CD pipelines for consistent results.
Quick Comparison: Mocking Strategies
Strategy | Best For | Setup Complexity | Speed | Testing Capabilities | Resource Usage |
---|---|---|---|---|---|
Mock Servers | Team collaboration, CI/CD | Medium | High | Handles complex scenarios | Medium |
Dynamic Response Rules | Stateful interactions, workflows | High | Medium | Advanced patterns | Low |
Local Files | Rapid prototyping, offline work | Low | High | Basic static responses | Minimal |
HTTP(S) Traffic Monitoring | Debugging, performance tests | Medium | Medium | Live traffic inspection | Low |
Test Automation | CI/CD, regression testing | High | Medium | Repeatable, automated tests | Medium |
Ready to save time and simplify your API development? Dive into the article to learn how to set up and use these methods effectively.
Why Use Request Mocking
Request mocking streamlines the development process, making it easier to integrate APIs efficiently while saving time and resources.
Speed Up Development Cycles
Using mock APIs allows teams to work in parallel without waiting for live APIs to be ready. This approach enables quick prototyping and iteration while letting team members maintain their own workflows. The result? Faster development and the ability to test edge cases more effectively.
Test Complex Scenarios
Mock APIs give developers full control over creating testing conditions that might be hard to reproduce with live services. You can simulate scenarios like authentication failures (401 Unauthorized), server errors (400 Bad Request or 500 Internal Server Error), or even network issues like slow connections and timeouts.
Cut Infrastructure Costs
By replacing live API calls with mock APIs, teams can avoid fees and usage limits during extensive testing phases, keeping costs down.
5 Methods to Mock API Requests
Set Up Mock Servers
Dedicated mock servers allow frontend teams to work independently by simulating backend APIs. You'll need to define key parameters like HTTP methods, Content-Type headers, response codes, and even add simulated delays to mimic real-world conditions.
To make your mock server more realistic, match your production API's URL patterns, validation rules, and response templates. You can even include custom headers if needed. As your testing scenarios get more complex, adjust responses dynamically to reflect those changes.
Create Dynamic Response Rules
Dynamic response rules let your mock server respond based on the details of incoming requests. This makes it possible to simulate complex backend behavior and state-dependent responses. For instance, a login API could handle various scenarios like:
- 200 OK: Returns user data for correct credentials.
- 401 Unauthorized: Indicates an incorrect password.
- 403 Forbidden: Denotes a locked account.
- 500 Internal Server Error: Simulates general server issues.
Once your mock server is set up, you can focus on monitoring and debugging your API traffic.
Monitor and Debug HTTP(S) Traffic
Real-time traffic monitoring is essential for debugging and verifying API behavior. This includes capabilities like:
- Viewing request and response headers.
- Inspecting detailed payload data.
This step helps identify and resolve integration issues quickly.
Use Local Files as Responses
Local files can act as API responses, making development faster and more efficient. This approach works well for:
- Large payloads.
- Binary data, such as images or documents.
- Complex JSON structures.
- Repeatedly used test cases.
To implement this, you’ll need to:
- Save response data in the proper file format.
- Configure headers like Content-Type to match the file type.
- Map file paths to specific API endpoints.
- Define appropriate response status codes.
Add Mocks to Test Automation
Integrating mocks into automated testing ensures consistent and reliable results. This is especially useful for:
- CI/CD pipelines.
- End-to-end tests.
- Performance testing.
- Validating error handling.
When setting up mocks for automated tests:
- Use version control for mock configurations.
- Maintain separate datasets for different environments.
- Initialize mocks at the start of tests.
- Clear or reset mock data after tests are completed.
Request Mocking Guidelines
Match API Specifications
Your mock endpoints should closely reflect the structure, validation rules, and response formats outlined in your API documentation. This ensures a smoother transition from development to production. Each mock endpoint should include:
- Response schemas
- HTTP status codes
- Header configurations
- Error handling patterns
- Version control mechanisms
Use Real-World Test Data
Using realistic test data can help simulate various user scenarios effectively. For example, a project saw a noticeable boost in email deliverability by incorporating diverse test data.
When creating mock data:
- Cover edge cases and boundary conditions
- Keep data relationships consistent across endpoints
- Update test datasets regularly to match new requirements
- Avoid using sensitive production data
Track Mock Configurations
Once your mock data and test setups align with live APIs, consistency in configuration becomes key. Document your mock setup thoroughly, including:
Configuration Aspect | Required Documentation | Update Frequency |
---|---|---|
Endpoint Mappings | URL patterns and response rules | Per API version |
Response Templates | Data structure and validation rules | As schemas change |
Test Scenarios | Coverage and expected behaviors | Sprint-based |
Environment Settings | Connection parameters and headers | Per deployment |
Store these mock configurations alongside your application code in version control. This approach ensures consistency and simplifies troubleshooting when issues arise. Regularly review and update your mock documentation to keep pace with changes in your API design and testing needs.
Mock Strategy Comparison Table
Pick a mocking strategy that aligns with your project's team size, complexity, and workflow. The table below breaks down key factors to help you make the right choice:
Mocking Strategy | Best For | Setup Complexity | Development Speed | Testing Capabilities | Resource Usage |
---|---|---|---|---|---|
Mock Servers | - Large teams - Continuous integration - Shared environments |
Medium | High – Simulates responses instantly | - Handles complex scenarios - Manages multiple endpoints - Supports state management |
Medium – Often cloud-based |
Dynamic Response Rules | - Edge case testing - Stateful interactions - Complex workflows |
High | Medium – Requires rule configuration | - Advanced patterns - Request sequences - Conditional responses |
Low – Local processing |
Local Files | - Rapid prototyping - Offline development - Simple endpoints |
Low | High – Quick to modify | - Basic scenarios - Static responses - Limited variations |
Minimal – File-based |
HTTP(S) Traffic Monitoring | - Debugging - Response analysis - Performance testing |
Medium | Medium – Real-time modifications | - Live traffic inspection - Response manipulation - Header/payload analysis |
Low – Local monitoring |
Test Automation Integration | - CI/CD pipelines - Regression testing - End-to-end testing |
High | Medium – Requires initial setup | - Automated scenarios - Repeatable tests - Validation rules |
Medium – Test environment |
Each strategy addresses specific needs: mock servers are great for team collaboration, dynamic rules handle intricate interactions, and local files are ideal for quick prototyping. Use this breakdown to match your mocking method to your development goals.
Key Factors to Consider:
- Infrastructure Needs: Mock servers may rely on cloud resources, while local files run directly on developer machines.
- Team Collaboration: Shared mock servers help maintain consistent testing across teams.
- Maintenance: Dynamic rules may require more upkeep but allow for greater testing flexibility.
- Workflow Fit: Local files are perfect for rapid iterations during early development.
- Testing Scope: More advanced strategies are better for edge case and comprehensive testing.
Conclusion
To wrap things up, integrating these practices can significantly improve your development process. Request mocking is a powerful way to speed up API development and feature delivery.
By removing backend dependencies, request mocking allows teams to work in parallel, speeding up development for projects of all sizes. Tools like mock servers and dynamic response rules let developers simulate detailed scenarios, test system resilience, maintain consistent environments, and cut down on infrastructure expenses.
This aligns with earlier recommendations, such as adhering to API specifications and using realistic test data. The key to success lies in choosing the right approach for your team - whether it's simple local file mocks or more advanced dynamic response rules. Picking the right strategy can make a big difference in your workflow.
Incorporating these methods can streamline the API development process and set the stage for ongoing improvements.