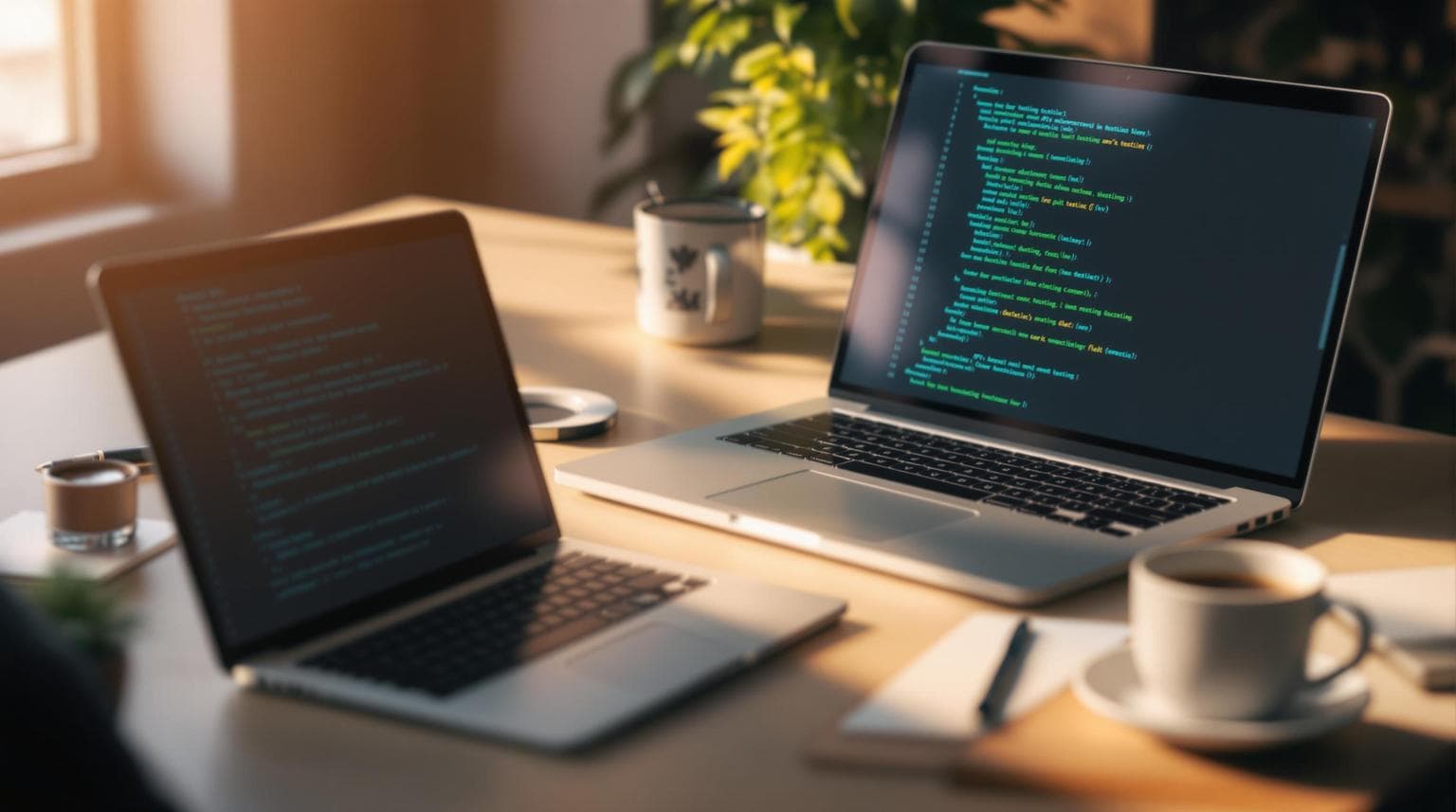
API Development Checklist: 10 Testing Essentials
Want reliable APIs? Start with this simple checklist. Testing ensures your APIs are functional, secure, and fast. Here's what to focus on:
- Validate Inputs and Outputs: Test data formats, status codes, and response times.
- Security: Check authentication, encryption, and rate limits.
- Error Handling: Ensure clear, actionable error responses.
- Performance: Measure speed, concurrency, and resource usage.
- Mock Server Testing: Simulate API behavior for controlled testing.
- Updates: Test backward compatibility and document changes.
- Version/Platform Testing: Ensure consistency across versions and devices.
- Edge Cases: Test limits like large payloads or timeouts.
- Usage Limits: Verify quota management and rate limits.
- Documentation and Logs: Keep everything clear and trackable.
Pro Tip: Use tools like ReqRes for real-time traffic analysis and debugging. Keep your APIs secure, fast, and user-friendly by testing regularly.
Getting Started with API Testing
Thorough preparation is essential for effective API testing. Here's how to lay the groundwork for a reliable testing environment.
Reading API Specs and Requirements
Begin by reviewing:
- API Documentation: Look into endpoint details, request/response structures, and authentication requirements.
- Data Format Standards: Check compliance with US formats, such as dates (MM/DD/YYYY) and currency ($).
- Performance Metrics: Identify expected response times and throughput limits.
- Security Measures: Understand authentication methods and encryption protocols.
"An API testing checklist acts as a comprehensive guide, helping developers and QA engineers systematically validate API functionality, performance, and security." - Patrick
Once you've reviewed the specs, gather and prepare test data to simulate real-world API scenarios.
Setting Up Test Data
Create test datasets that mimic actual API usage. Here's how:
Environment Configuration
Set up distinct environments for development, staging, and production. Each should have isolated test data to avoid interference.
Test Data Categories
Data Type | Purpose | Example Scenarios |
---|---|---|
Valid Input | Test normal operations | Complete user profiles, standard transactions ($10-$1,000) |
Edge Cases | Test boundaries | Maximum file sizes (100MB), character limits (255) |
Invalid Data | Check error handling | Malformed JSON, incorrect date formats |
Security Tests | Test access controls | Expired tokens, invalid credentials |
To make your test data more effective, consider these practical steps:
1. Design Realistic Scenarios
Include real-world use cases that reflect how the API will be used. Focus on typical data patterns and common user behaviors.
2. Validate Data Inputs
Test both valid and invalid inputs across all data types. For instance, when testing a user registration endpoint:
- Valid email formats: user@domain.com
- Invalid formats: user@domain, incomplete@.com
- Special characters in names: O'Connor, García
- Different phone formats: (555) 123-4567
3. Enable Monitoring and Logging
Activate detailed logging for API requests and responses. Tools like ReqRes (for macOS) can help inspect HTTP(S) traffic and troubleshoot effectively.
10 Must-Do API Tests
With proper preparation and test data in place, focus on these 10 essential tests to ensure your APIs are reliable, secure, and efficient.
1. Input and Output Testing
Start by validating the inputs and outputs of your API. Key areas to test include:
- Data Format: Ensure request and response payloads follow the expected structure and data types.
- Status Codes: Verify that the API returns correct HTTP status codes (e.g., 200 for success, 400 for client errors).
- Response Times: Measure response times under normal conditions to confirm timely data exchange.
Next, focus on securing your API.
2. Security Checks
Strengthen your API's defenses by testing the following:
Aspect | Focus | Action |
---|---|---|
Authentication | Token validation | Check token integrity and expiration |
Authorization | Role-based access | Verify permission hierarchies |
Data Protection | Encryption | Ensure data is encrypted during transit |
Rate Limiting | Request throttling | Confirm rate limits align with policies |
After security, ensure error responses are clear and actionable.
3. Error Response Testing
Test how your API handles errors by checking:
- Invalid Authentication: Verify that invalid or expired tokens return a 401 status.
- Missing Resources: Ensure non-existent endpoints return a 404 status.
- Rate Limits: Confirm that exceeding rate limits results in a 429 status, with retry details included.
4. Performance Measurement
Evaluate how well your API performs under different conditions:
- Measure response times to ensure they meet expectations.
- Test the API's ability to handle concurrent requests.
- Monitor resource usage, including CPU, memory, and network bandwidth.
5. Mock Server Testing
Use mock servers to simulate API behavior in controlled environments. This allows you to:
- Test request and response interactions.
- Simulate varying network conditions.
- Reproduce error scenarios to test resilience.
6. Update Testing
When rolling out updates:
- Run regression tests to confirm that existing functionality remains intact.
- Ensure backward compatibility for older versions.
- Clearly document all changes in a changelog.
7. Version and Platform Testing
Test across different versions and platforms to maintain consistency:
- Validate functionality across API versions.
- Check compatibility with various client platforms, including mobile and web interfaces.
- If applicable, test browser compatibility.
8. Edge Case Testing
Push your API to its limits by testing edge cases:
- Handle large payloads to assess performance.
- Verify proper character encoding in requests and responses.
- Simulate timeouts to evaluate error handling.
9. Usage Limit Testing
Ensure your API adheres to usage limits:
- Test how it responds to bursts of requests.
- Monitor quota management and enforcement.
- Confirm that rate limit headers are correctly implemented in responses.
10. Documentation and Logs
Keep your API well-documented. Include detailed specifications, authentication guidelines, and error code explanations. Accurate logs are also essential for troubleshooting and monitoring.
macOS Debug Tools Guide
Expand your API testing capabilities on macOS by using tools designed to inspect and debug HTTP(S) traffic.
Using ReqRes for Traffic Analysis
Simplify API testing on macOS with ReqRes's built-in tools for inspecting HTTP(S) traffic.
Feature | Functionality | Example Use Case |
---|---|---|
Real-time Monitoring | Track HTTP(S) requests live | Debugging API calls |
Mock Endpoints | Simulate API responses | Testing client behavior |
Local File Mapping | Serve local files as responses | Supporting local development |
Traffic Interception | Inspect HTTP(S) traffic | Troubleshooting issues |
Getting started with ReqRes is quick and straightforward:
- Install ReqRes on your macOS device.
- Enable traffic inspection during setup.
- Begin your API testing session.
- Use the interface to view and analyze requests and responses.
Traffic Debug Tips
After setting up, these tips can help you fine-tune your traffic analysis:
Request and Response Validation
- Inspect headers, payloads, and request formats in real-time.
- Confirm authentication tokens and query parameters.
- Match status codes with API standards.
- Track response times and performance metrics.
Advanced Features
- Use local file mapping to test responses.
- Set up mock endpoints to handle edge cases.
- Group related requests for better organization during debugging.
The macOS interface makes it easier to manage and analyze API traffic. For teams, ReqRes supports up to 5 devices under the Team Plan, enabling collaborative debugging across multiple developers.
Conclusion
A solid API testing checklist ensures APIs are secure and function as expected. Automated tools make validation easier and help identify issues faster. Consistent API testing not only speeds up development but also ensures problems are caught early, minimizing their impact on users.
ReqRes's macOS interface supports this process with features like:
Testing Aspect | Benefit to Development |
---|---|
Real-time Monitoring | Instant insights into API behavior and performance |
Traffic Analysis | Quick detection of request/response problems |
Mock Endpoints | Speeds up development with simulated responses |
Local File Mapping | Simplifies testing for complex scenarios |
These tools create a smoother, more efficient testing workflow, helping teams stay ahead of potential issues.
To keep your APIs reliable:
- Run security scans on a regular basis
- Track performance metrics consistently
- Log critical interactions for analysis
- Regularly review and refine testing outcomes