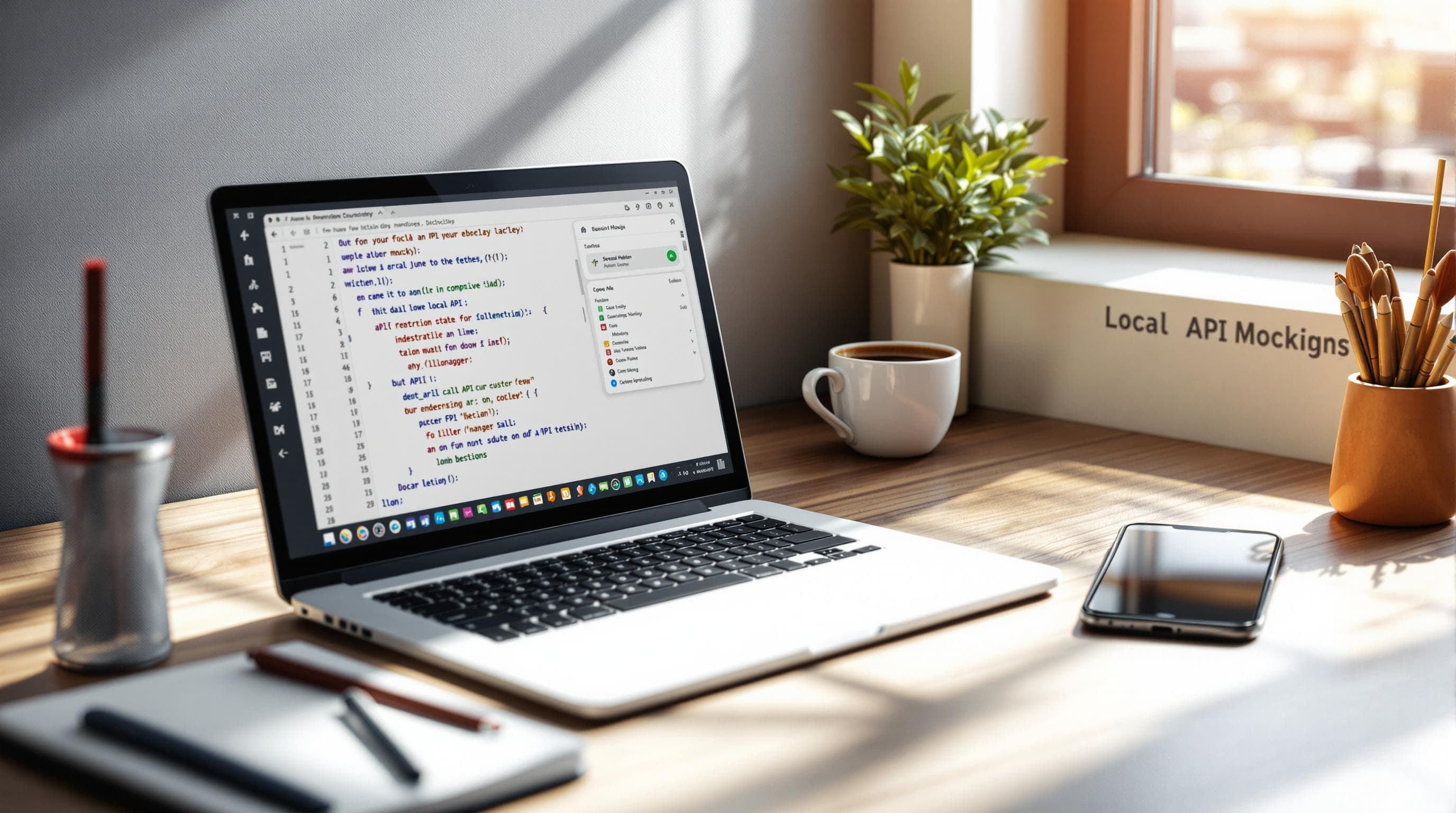
Local API Mocking: A Step-by-Step Guide for Developers
Nearly 90% of developers rely on APIs, and local API mocking can save time, cut costs, and improve testing. It lets you simulate API responses without live backend systems, enabling faster development and debugging. Here's why it matters:
- Types of Mocking: Static (fixed responses), Dynamic (input-based), Proxy (record/replay).
- Key Benefits: Improved security, parallel workflows, unlimited testing, and consistent debugging.
- Tool Spotlight: ReqRes for macOS simplifies API mocking with features like dynamic templates, traffic monitoring, and SSL support. Setup costs $59.99/year.
Quick Setup for ReqRes: Download, adjust system settings, and configure mock endpoints. Test with tools like curl or API clients to ensure everything works.
Mocking helps frontend teams build UIs without backend dependencies and lets backend teams validate services in isolation. It’s also useful for load testing by simulating latency, high traffic, or errors.
Mocking Scenarios:
- Frontend: Test UI responses for success, errors, and timeouts.
- Backend: Validate service interactions, error handling, and data processing.
- Load Testing: Simulate network conditions like high latency or random failures.
ReqRes also supports advanced features like dynamic response templates and real-time traffic monitoring, making it ideal for both small and large projects.
Tip: Keep mock configurations in version control and align them with production APIs for accurate testing.
Local API mocking is a game-changer for developers, enabling faster, safer, and more efficient workflows.
Setting Up ReqRes for API Mocking
System Requirements
ReqRes works seamlessly on macOS with minimal resource demands. Ensure your system meets these requirements:
- macOS 11.0 (Big Sur) or later
- At least 4GB of RAM
- 500MB of free storage
- Admin privileges for installation
- Internet access for updates
Installation Steps
Here’s how to get started:
- Download and Install Head over to reqresapp.com and download the latest version. A one-time payment of $59.99 covers a year of updates. Once downloaded, open the .dmg file and drag the ReqRes app into your Applications folder.
-
Adjust System Settings
Go to System Preferences > Security & Privacy > Privacy tab. Make sure to enable ReqRes access under these categories:
- Network
- Full Disk Access
- Developer Tools
-
Complete Initial Setup
Open ReqRes and go through the setup wizard. The app will automatically adjust your system’s proxy settings and install the required SSL certificate.
Feature Default Setting Recommended Setting Port Number 8080 8080 (or any available port) SSL Inspection Disabled Enabled Auto-save Responses Enabled Enabled Response Delay 0ms 200ms (for realistic latency)
Verifying Mock API Setup
Set up a mock endpoint like GET /api/users
, define a sample JSON response, and test it using ReqRes’s built-in tools, curl, or your preferred API client.
If everything is configured correctly, you’ll see your mock response, complete with the correct HTTP status code and headers. Use the ReqRes traffic inspector to confirm the request-response cycle.
For best results, keep detailed documentation of your mock API configurations. Include endpoint paths, request methods, and expected responses. This helps maintain consistency across your team and makes troubleshooting easier. With these steps, you’re ready to dive into various API mocking scenarios.
Common API Mocking Scenarios
With ReqRes set up, you can simplify both frontend and backend testing by simulating real API behavior in various situations.
Frontend Development Tasks
Frontend teams can build and test user interfaces quickly, even when the backend isn't ready, by using simulated API responses.
Response Type | Mock Configuration | Testing Scenario |
---|---|---|
Success (200) | Valid user data | Display user profile |
Error (404) | Empty response | Show "User not found" message |
Error (500) | Server error message | Test error handling |
Timeout | 5000ms delay | Validate loading indicators |
For example, you can mock responses for login attempts, password resets, account verification, or session timeouts. This helps ensure the UI responds correctly in various situations.
Backend Testing Methods
Mock APIs are just as useful for backend testing, offering a controlled environment to isolate and validate specific components without relying on live services.
This approach allows you to:
- Simulate interactions between services
- Test how errors are handled
- Verify data transformation processes
- Check authentication mechanisms
For larger systems, you can maintain separate mock configurations for different scenarios. This ensures you can thoroughly test business logic without needing external dependencies.
Load Testing Options
Using mock APIs for load testing helps you evaluate how your application performs under different conditions. You can configure your mock environment to simulate various network scenarios:
Test Scenario | Configuration | Purpose |
---|---|---|
High Latency | 500ms delay | Test timeout handling |
Bandwidth Limit | 1 Mbps cap | Verify data streaming behavior |
Request Volume | 1000 requests/second | Assess performance under load |
Random Failures | 5% error rate | Test system resilience |
Start with basic responses, then introduce delays, errors, or high request volumes to mimic real-world conditions. Keep an eye on system resources during these tests to ensure your mock API server remains stable.
Mock APIs provide a cost-effective, controlled environment, making them an excellent tool for startups and development teams alike.
ReqRes Advanced Features
Creating Dynamic Response Templates
ReqRes includes a powerful templating system to help you create dynamic and realistic responses for testing. This system enables features like generating random user profiles, adjusting responses based on request parameters, applying conditional logic, and creating data loops.
Template Feature | Purpose | Example |
---|---|---|
Random Data | Generate user profiles | Names, emails, addresses |
Request Parameters | Adjust responses | Adapt to query parameters and headers |
Conditional Logic | Status-specific data | Return different outputs based on auth state |
Data Loops | Build collections | Create lists with varying lengths |
Here’s an example of how you can use these tools to design a dynamic response:
{
"users": {{repeat 5}},
"name": "{{faker 'name.fullName'}}",
"email": "{{faker 'internet.email'}}",
"created_at": "{{date 'MM-DD-YYYY'}}"
}
Once you've set up your templates, you can assess their effectiveness by monitoring live traffic and ensuring everything works as intended.
Traffic Monitoring and Control
ReqRes allows you to monitor HTTP(S) traffic in real time. This feature helps you review detailed interaction data, making it easier to confirm that your mock APIs are functioning correctly without needing live servers. This functionality also simplifies team collaboration by providing clear insights into API behavior.
Team Usage Guidelines
Here are some tips for using ReqRes effectively as a team:
- Configuration Management: Keep your mock API definitions in version control for better tracking and collaboration.
- Access Control: Protect your mock server with API keys to manage access securely.
- Device Synchronization: Share configurations and settings across all team members’ devices for consistency.
- Update Coordination: Ensure everyone is working with the same mock data to avoid discrepancies.
The team plan is designed for small to medium-sized teams, supporting up to 5 devices with a one-time cost of $149.99. Need more devices? Add them for $5 each. Annual renewals are $24.99 to keep your setup updated and supported.
API Mocking Guidelines
Matching Production APIs
Clearly define production endpoints, error codes, headers, and latency early in the process - ideally during the design phase. This helps validate assumptions before development kicks off.
Aspect | Implementation Strategy | Validation Method |
---|---|---|
Response Format | Replicate the exact JSON/XML structure | Automated schema validation |
Status Codes | Cover all possible production error states | Edge case testing |
Headers | Include authentication tokens | Security verification |
Latency | Mimic realistic response times | Performance monitoring |
Once you’ve aligned your mock APIs with production, manage your mock data carefully to ensure it stays consistent and accurate.
Mock Data Management
- Save mock configurations in your version control system.
- Provide thorough documentation for mock scenarios and their expected behaviors.
- Use dynamic responses based on input parameters to handle variability.
- Implement stateful mocking for workflows that require multiple steps.
These steps help ensure your mock data remains reliable as your APIs grow and change.
Maintaining Mock Accuracy
To keep your mocks aligned with production APIs, follow these practices and routinely validate them as changes are made:
1. Contract Testing Integration
Validate service interactions to ensure they comply with your API specifications.
2. CI/CD Pipeline Integration
Automate mock response checks with every code update to catch discrepancies early.
3. Documentation Updates
Keep detailed records of mock configurations, scenarios, and any known limitations to avoid confusion.
For more complex workflows, use realistic test data to better simulate production scenarios:
Scenario Type | Mock Implementation | Validation Method |
---|---|---|
High Load | Simulate multiple concurrent requests | Load testing tools |
Error States | Create specific error responses | Error handling verification |
Edge Cases | Test boundary conditions | Integration testing |
Security | Mock authentication and authorization flows | Security testing suite |
Conclusion
Summary
API mocking plays a crucial role in modern development, with nearly 90% of developers relying on APIs. By using local API mocking, teams can work independently of live servers, speeding up workflows and cutting costs tied to third-party APIs.
Benefit | Impact |
---|---|
Development Speed | Enables parallel workflows |
Cost Efficiency | Avoids third-party API expenses |
Testing Reliability | Provides a stable, controlled setup |
Security | Lowers the risk of data breaches |
ReqRes Benefits
ReqRes offers a powerful solution for macOS developers managing high request volumes. This native macOS app combines advanced traffic monitoring with a reliable 99.99% uptime SLA on Heroku.
Key features of ReqRes include:
- CORS-enabled API: Handles requests from any domain seamlessly.
- Support for HTTP methods: Simulates real-world scenarios, including authentication.
- Customizable response delays: Perfect for mimicking loading states.
- Zero data storage policy: Ensures compliance with security standards.
With its straightforward setup and user-friendly design, ReqRes simplifies rapid prototyping and streamlines development workflows. Its comprehensive feature set is ideal for handling complex testing needs and creating production-like simulations.