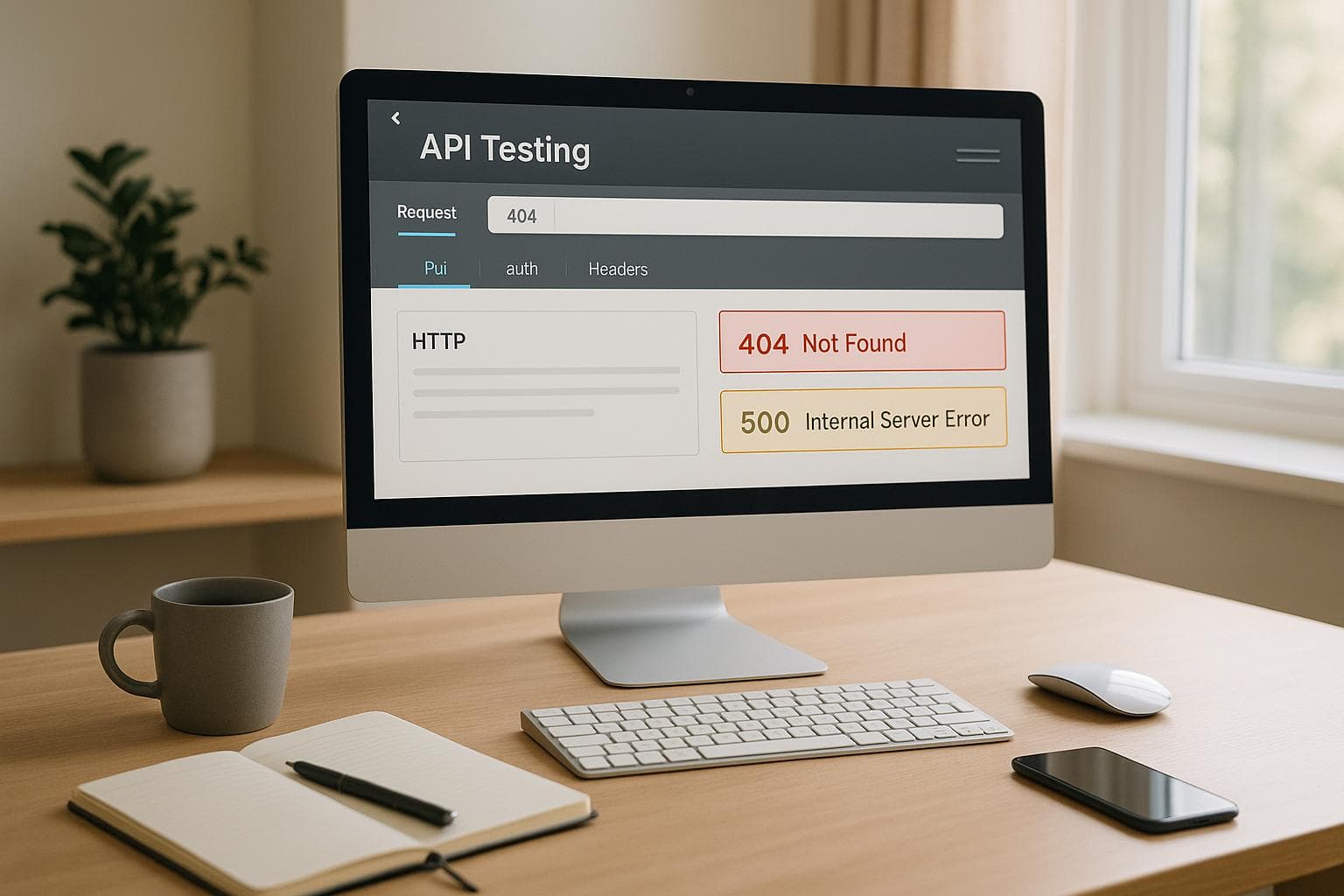
Simulating HTTP Errors: Best Practices for API Testing
Simulating HTTP errors is crucial for ensuring APIs can handle issues effectively. Here's what you need to know:
- Why It Matters: Testing HTTP errors helps validate error handling, test edge cases, and ensure APIs meet standards.
- Key Errors to Test:
- 4xx (Client Errors): Test invalid requests like
400 Bad Request
,401 Unauthorized
, or404 Not Found
. - 5xx (Server Errors): Simulate server issues like
500 Internal Server Error
or503 Service Unavailable
.
- 4xx (Client Errors): Test invalid requests like
- How to Test:
- Use tools like ReqRes for endpoint mocking and local file mapping.
- Simulate scenarios like timeouts, outages, and malformed data.
- Best Practices:
- Automate testing workflows.
- Maintain consistent error responses.
- Use mock tools to replicate production conditions safely.
Quick Comparison of Testing Methods:
Method | Setup Time | Flexibility | Best Use Case | Limitations |
---|---|---|---|---|
Manual Server Changes | 2-4 hours | Low | Production validation | Requires server access, high risk |
Local Mock Servers | 30-60 mins | Medium | Development testing | Limited to basic scenarios |
Automated Tools | 15-30 mins | High | Continuous integration | Requires initial configuration |
ReqRes Implementation | 5-10 mins | Very High | Development & testing | macOS-only compatibility |
Simulating errors ensures your API is reliable and resilient, minimizing risks before deployment.
Common HTTP Error Types
Knowing HTTP error codes is essential for effective API testing. These codes help developers figure out whether issues stem from the client’s request or the server’s operations.
4xx Client Errors
Client errors happen when the request sent by the client is problematic. These issues usually need to be fixed on the client side.
Key 4xx errors to test include:
- 400 Bad Request: The server can’t process the request due to bad syntax or an invalid request format.
- 401 Unauthorized: The request lacks valid authentication credentials.
- 403 Forbidden: The server understands the request but refuses to authorize it.
- 404 Not Found: The requested resource isn’t available on the server.
- 429 Too Many Requests: The client has exceeded the allowed number of requests.
Important considerations for handling these errors:
- Show clear, user-friendly error messages.
- Manage authentication issues effectively.
- Offer clear steps for resolving errors.
- Ensure the system remains stable during error responses.
Server-side problems, however, require a different testing approach.
5xx Server Errors
Server errors indicate issues on the server side and often demand backend troubleshooting. These errors can disrupt the experience for all users.
Key 5xx errors to simulate include:
- 500 Internal Server Error: A generic issue signaling a server malfunction.
- 502 Bad Gateway: Happens when an upstream server returns an invalid response.
- 503 Service Unavailable: The server is temporarily unable to handle requests.
- 504 Gateway Timeout: The server waits too long for a response from an upstream server.
Best practices for managing these errors include:
- Implement fallback solutions to minimize disruptions.
- Retry failed requests when suitable.
- Ensure data remains consistent during server issues.
- Provide users with clear, helpful feedback during outages.
Error Category | Focus Area | Testing Priority |
---|---|---|
4xx Errors | Request validation & client behavior | High – These are common in production |
5xx Errors | System resilience & recovery | Critical – These impact all users |
Tools like ReqRes make it easier to simulate these error scenarios through endpoint mocking. This allows developers to test error handling without needing a live server, ensuring both client and server errors are tested thoroughly in a controlled setup.
These distinctions help shape focused testing strategies, which are explored further in the next section.
Error Simulation Guidelines
This section outlines methods to simulate and test common HTTP errors, helping you assess your system's error handling and overall stability.
Testing Invalid Requests
Here are some scenarios to test for invalid requests:
- Send malformed JSON to provoke error responses.
- Use invalid or missing parameters to check error handling.
- Test how the system reacts to unauthorized requests.
- Verify rate limit enforcement under heavy request loads.
You can simulate these errors effectively by using endpoint mocks. Testing beyond invalid requests - like mimicking real-world production issues - can further enhance your API's resilience.
Simulating Production Errors
The table below highlights common error scenarios and how to test them:
Error Scenario | Testing Approach | Expected Outcome |
---|---|---|
Network Timeouts | Simulate delayed responses | Ensure retry mechanisms work |
Service Outages | Mock complete service unavailability | Test fallback solutions |
Data Corruption | Return malformed response data | Confirm proper error parsing |
Partial System Failures | Mock failures in specific endpoints | Check system resilience |
Tools like ReqRes can help you simulate these scenarios effectively.
Using Mock Testing Tools
ReqRes simplifies error simulation by providing features that create dependable test environments:
- Endpoint Mocking: Set up mock endpoints to return specific error responses.
- Local File Mapping: Modify response content using local files.
- Traffic Interception: Monitor HTTP(S) requests and responses in plain text.
When using mock testing tools, follow these steps:
- Design test cases that closely replicate production conditions.
- Ensure mocked responses follow the API's format.
- Leverage local file mapping to test edge cases.
These tools and techniques help you test edge cases thoroughly and maintain consistent error responses across your system.
Error Simulation Tools
Specialized tools can improve the precision of error testing. One such tool, ReqRes, allows you to simulate HTTP errors effectively.
ReqRes Error Testing Features
ReqRes provides several capabilities for monitoring and debugging HTTP(S) traffic:
- Intercept and monitor real-time HTTP(S) traffic in plain text.
- Set up mock endpoints to return specific error responses.
- Analyze and debug error scenarios with detailed insights.
For even more efficient testing, you can use local file mapping to manage error responses.
Local File Response Mapping
Local file mapping helps you test error responses quickly and efficiently. Here's how it works:
-
Create Error Response Files
Prepare local JSON files that define error payloads. For instance:{ "status": 503, "error": "Service Unavailable", "message": "System maintenance in progress" }
-
Configure Response Rules
Use ReqRes to set up rules that map specific requests to these local files. This allows you to test error responses without altering your server. -
Validate Error Handling
Test how your application reacts to these predefined error scenarios, ensuring it handles issues as expected.
Test Automation Options
Automation can streamline error simulations by making responses more consistent and predictable.
Component | Approach | Advantages |
---|---|---|
Response Templates | Build reusable templates | Ensures uniform testing |
Request Matching | Define specific trigger rules | Creates reliable tests |
Traffic Monitoring | Automate validation processes | Speeds up debugging |
Testing Method Analysis
Comparing Testing Methods
Error simulation methods differ in effectiveness depending on specific testing needs. Factors like setup time and ease of use can significantly impact the efficiency of your testing process.
Testing Method | Setup Time | Flexibility | Best Use Case | Limitations |
---|---|---|---|---|
Manual Server Changes | 2-4 hours | Low | Production validation | Requires server access; higher risk |
Local Mock Servers | 30-60 mins | Medium | Development testing | Limited to basic scenarios |
Automated Tools | 15-30 mins | High | Continuous integration | Requires initial configuration |
ReqRes Implementation | 5-10 mins | Very High | Development & testing | macOS-only compatibility |
These methods cater to different stages of production and development. While traditional techniques demand more time and effort, modern solutions like ReqRes simplify the process with faster setup and greater flexibility. Let’s dive into how ReqRes redefines error simulation with its macOS-focused features.
Features of ReqRes for Testing
ReqRes simplifies error simulation with tools designed for speed and precision. It eliminates the need for server alterations by offering features like real-time traffic analysis, advanced endpoint mocking, and local response mapping.
Real-Time Traffic Analysis
- Quickly spot error patterns.
- Debug response handling as it happens.
- Check error message formatting on the fly.
Advanced Mocking Capabilities
- Simulate complex error scenarios.
- Maintain consistent testing environments.
Local Response Mapping
ReqRes’s Map Local Tool is particularly useful for error testing:
Feature | Benefit | Implementation |
---|---|---|
File-Based Responses | Test various scenarios | Map requests to local JSON files |
Conclusion
Summary
Simulating HTTP errors helps developers create applications that can handle unexpected issues effectively. Using efficient tools for error simulation simplifies testing and reduces complexity.
In API testing, tools like ReqRes have made a noticeable impact. For instance, ReqRes's Map Local Tool allows developers to use local files as mock responses, streamlining the testing process.
API testing tools continue to advance, offering features like SSL web server traffic logging and plain-text displays of HTTP(S) requests and responses. These features help developers catch potential problems early in the development process.
Here are some key benefits of modern error simulation tools:
- Simplified Setup: Quickly configure error scenarios and focus more on testing.
- Comprehensive Testing: Simulate both 4xx and 5xx errors to ensure robust application behavior.
- Traffic Analysis: Monitor HTTP(S) traffic to improve debugging and pinpoint issues.
To make the most of these tools, consider these strategies:
- Automate testing workflows to include error scenarios.
- Use local file response mapping for quick testing of edge cases.
- Maintain consistent testing environments throughout development.
- Utilize real-time traffic analysis to get immediate insights.
As APIs play a growing role in modern applications, thorough error testing is essential. By following these best practices and using the right tools, teams can build more reliable and resilient applications.
FAQs
Why is simulating HTTP errors important for improving API reliability before deployment?
Simulating HTTP errors is essential for ensuring your API is reliable and resilient before it goes live. By testing how your API responds to various error scenarios, such as server outages or invalid requests, you can identify weaknesses and address them early in development.
This process helps ensure your API can handle unexpected situations gracefully, reducing downtime and improving user experience. Proactive testing leads to a more robust and dependable API, ready to perform under real-world conditions.
What are the benefits of using ReqRes for simulating HTTP errors instead of modifying the server manually?
Using ReqRes for simulating HTTP errors provides a more efficient and flexible approach compared to manually altering server configurations. As a macOS application, ReqRes allows you to monitor, debug, and mock HTTP(S) traffic in real-time, enabling seamless testing without needing to deploy or modify the actual API.
With features like endpoint and server mocking, you can simulate various error scenarios quickly, saving time and effort during development. Additionally, the Map Local Tool lets you use local files as responses, making it easier to test edge cases or unexpected behaviors without affecting server data. This streamlined process helps ensure more reliable and thorough API testing.
How can ReqRes help simulate 4xx and 5xx HTTP errors for better API testing?
ReqRes simplifies API testing by allowing developers to mock endpoints and simulate 4xx and 5xx HTTP error responses. With its real-time request interception and response customization features, you can test error scenarios without relying on a live API. This is especially helpful for identifying edge cases, ensuring robust error handling, and streamlining development workflows.