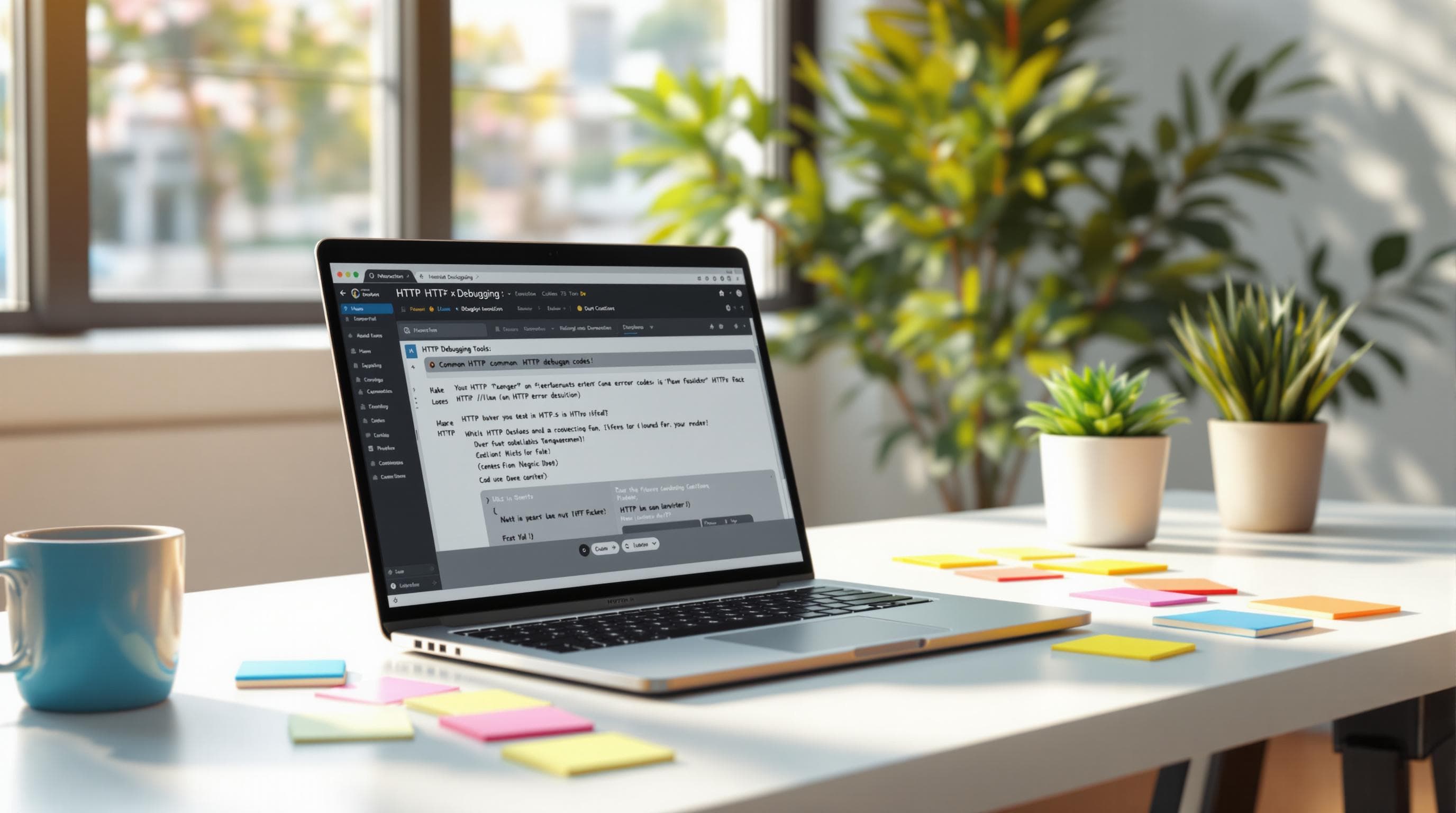
8 Common HTTP Debugging Problems and Solutions
- Can’t Monitor Traffic? Check proxy settings, disable VPNs, or map localhost to a domain.
- HTTPS Inspection Issues? Install trusted SSL certificates and configure SSL proxying.
- Struggling to Find Requests? Use URL patterns, headers, or content type filters.
- Testing Edge Cases? Mock responses like 404 errors or malformed JSON locally.
- Performance Problems? Analyze response times, enable compression, and optimize queries.
- CORS Errors? Configure
Access-Control-Allow-*
headers correctly to avoid browser restrictions. - Testing Multiple Endpoints? Capture, replay, and validate requests with tools like HAR files.
- Team Collaboration? Share debugging setups and sync environments for consistency.
Quick Comparison
Problem | Solution | Tools/Actions |
---|---|---|
Unable to Monitor Traffic | Fix proxy settings, disable VPNs, map localhost | ReqRes, Map Local |
HTTPS Traffic Issues | Install SSL certificates, configure proxying | Keychain Access, SSL Proxy Settings |
Finding Specific Requests | Use filters (URL, headers, content type) | Templates for filtering |
Testing API Edge Cases | Mock responses, test locally | Local JSON files, response mapping |
API Performance Issues | Check response times, optimize resources | Gzip, HTTP/2, caching, query analysis |
Fixing CORS Errors | Set proper headers (Access-Control-Allow-* ) |
Browser dev tools, header configuration |
Testing Multiple Endpoints | Capture, replay, validate | HAR files, Postman, session storage |
Team Debugging Setup | Share settings, sync devices | Rule lists, workspace sharing |
Start with these fixes to streamline your debugging process and improve your workflow.
1. Unable to Monitor HTTP(S) Traffic
Developers often encounter a frustrating issue: their HTTP debugging tool fails to capture network traffic. This problem usually arises from incorrect system configurations.
Quick Setup with ReqRes
Here’s how to ensure reliable HTTP traffic capture:
- System Proxy Configuration: Make sure your HTTP proxy is set to 127.0.0.1 with the correct port. If the request list is empty, it’s likely a proxy configuration issue.
- VPN Interference: Active VPNs can disrupt HTTP proxy settings, blocking traffic from routing through the debugging proxy. To fix this:
- Turn off any active VPN connections.
- Recheck your system proxy settings after disconnecting the VPN.
- Restart your debugging session.
- Localhost Traffic on macOS: macOS often bypasses proxy settings for localhost. Fix this by mapping localhost to a domain in
/etc/hosts
or using a fully qualified domain name.
Once configured, verify that traffic is being captured properly. Use the Map Local feature to simulate responses instantly for quick testing.
Map Local for Quick Testing
The Map Local feature allows you to create mock responses instantly, eliminating the need for a full test server. This is a quick way to ensure your traffic monitoring setup is functioning correctly.
Common Setup Issues
Issue | Solution | Impact |
---|---|---|
Empty Request List | Check proxy settings and disable VPN | Enables traffic capture |
Cached Requests | Turn off caching in the HTTP client | See all requests in real time |
Remote Device Connection | Ensure devices are on the same network and required ports are open | Allows mobile testing |
If you’re working on a company network, proxy ports might be blocked. Coordinate with your IT team to open the necessary ports, allow incoming connections, and adjust firewall settings.
For iOS 16-specific problems, try disconnecting and reconnecting to the network, testing with an iOS 15 simulator, or using alternative debugging tools.
These steps will help ensure your setup is ready for smooth and effective HTTP debugging.
2. HTTPS Traffic Inspection Issues
When dealing with HTTPS traffic, extra steps are required due to encryption. Inspecting HTTPS traffic can be tricky because of its built-in security features.
SSL Certificate Setup
To inspect HTTPS traffic, you need to install and configure SSL certificates. Here's how:
-
Install the Root Certificate
- Go to Help > SSL Proxying > Install Charles Root Certificate.
- Move the certificate from the "login" keychain to the "System" keychain.
- Ensure the certificate is marked as "trusted" in Keychain Access.
-
Configure SSL Proxying
- Right-click the target URL in the request list or go to Proxy > SSL Proxying Settings to enable SSL Proxying for specific domains.
SSL-Specific Issues | Solution | Impact |
---|---|---|
Certificate Not Trusted | Move to System keychain | Enables HTTPS inspection |
SSL Handshake Failures | Verify certificate trust | Prevents connection errors |
SSL Pinning Blocks | Temporarily disable SSL pinning | Allows traffic inspection |
Request Data Analysis
Once SSL is configured, you can start analyzing decrypted data. Focus on key areas like:
- Headers: Look for authentication details.
- Cookies: Check session management.
- Payloads: Examine the data format being used.
For some frameworks (e.g., Ruby, NodeJS, Python, Golang), additional setup may be needed to trust self-signed certificates.
Troubleshooting Tips:
- Update
network_security_config.xml
andAndroidManifest.xml
for Android apps to trust the debugging certificate. - For React Native and Flutter, follow platform-specific instructions to establish certificate trust.
- Configure the JVM to recognize the debugging certificate for Java applications.
3. Finding Specific HTTP Requests
Narrow down HTTP requests by applying targeted filters.
Filter HTTP Traffic
Use these filtering methods to pinpoint specific requests:
-
URL Pattern Matching: Set URL filters to target specific endpoints. For example:
https://api.example.com/auth/*
-
Content Type Filtering: Focus on specific content types with these filters:
Content Type Example Filter application/json
Content-Type: application/json
multipart/form-data
Content-Type: multipart/form-data
application/x-www-form-urlencoded
Content-Type: application/x-www-form-urlencoded
-
Header-Based Filtering: Target headers to capture tokens, custom headers, or correlation IDs, such as:
Authorization: Bearer * X-Request-ID: *
Save these filters as templates for quicker debugging in the future.
Create Filter Templates
Reusable templates can simplify debugging by combining multiple filters.
-
Request Templates: Include these elements for precise filtering:
- HTTP methods (e.g., GET, POST, PUT, DELETE)
- URL patterns
- Header combinations
- Request body formats
-
Response Templates: Focus on:
- Status codes (e.g., 2xx, 4xx, 5xx)
- Specific response headers
- Patterns in the response body
Tip: For microservices, maintain separate template sets for each service to keep your debugging process organized.
Template Type | Use Case | Key Components |
---|---|---|
API Authentication | Track auth flows | Method, Auth headers, Tokens |
Error Tracking | Monitor failures | 4xx/5xx status, Error responses |
Performance | Identify slow requests | Response time, Payload size |
To quickly isolate issues, combine filters in a single template. For example:
Method: POST
URL: */auth/*
Headers: Authorization, X-API-Key
Status: 401, 403
This setup helps identify authentication problems efficiently.
4. Testing API Edge Cases
Strengthen your application's reliability by simulating a variety of API responses. Testing both normal and unexpected situations helps ensure your API can handle anything thrown its way.
Create Mock API Responses
Simulating different API responses is key to testing how well your application handles errors. Focus on key status codes like 404 (not found), 429 (rate limiting), and 500 (server error). Go further by creating dynamic templates to test scenarios such as:
- Malformed JSON
- Missing or extra fields
- Empty data sets
- Handling special characters
These tests help you uncover potential weak points in your error-handling logic.
Local File Response Testing
Using local files for API responses allows you to test without relying on external systems. This method ensures a controlled environment for testing.
How to Set Up Local File Responses:
- Create local JSON files for your test cases.
- Set up proper response headers (like
Content-Type
). - Map specific API requests to these local files.
- Test how your application handles each scenario.
For example, you can use a local file to simulate a 404 error, allowing you to confirm fallback behavior without touching live APIs.
Tips for Effective Local Testing:
- Keep test files organized in a clear directory structure.
- Use version control to track changes in your test data.
- Document the expected outcomes for each test case.
- Include a mix of valid and invalid data in your tests.
- Experiment with varying response times to mimic real-world delays.
Testing edge cases like these ensures your application stays stable, even in unpredictable situations.
5. Finding API Performance Issues
API performance problems can hurt both responsiveness and reliability, so spotting and fixing them is crucial.
Check Response Times
Keep an eye on API response times to identify where delays occur. Focus on these metrics:
- Time to First Byte (TTFB): Measures how quickly the server sends the first byte of data.
- Total Response Time: Tracks the full time it takes to complete the request-response cycle.
- Error Rates: Shows how often requests fail.
For high-priority applications, aim for response times between 100–500ms. For less critical tasks, 5–15 seconds might be acceptable.
To improve response times, consider:
- Using Gzip or Brotli compression to shrink payload sizes.
- Upgrading to HTTP/2 for faster data transfers.
- Setting up caching for frequently requested data.
- Optimizing database queries with execution plan analysis.
Additionally, enable detailed logging, establish performance baselines, analyze slow responses, and monitor memory and CPU usage. Comparing metrics across environments can also reveal context-specific issues.
Test Different Environments
Testing performance in various environments - development, staging, and production - helps fine-tune optimization efforts. Use tools like load testing, network latency simulation, and resource monitoring to assess performance under different conditions.
Here’s an example load test configuration using Artillery CLI:
config:
target: 'https://api.example.com'
phases:
- duration: 60
arrivalRate: 100
scenarios:
- flow:
- get:
url: '/v1/products'
You can also use the delay parameter to simulate timeouts and loading behaviors:
?delay=2
Track these key metrics across environments:
Metric | Target Range | Warning Threshold |
---|---|---|
Response Time | < 500ms | > 1000ms |
Error Rate | < 0.1% | > 1% |
CPU Usage | < 70% | > 85% |
Memory Usage | < 75% | > 90% |
Set up alerts for any metrics that exceed warning thresholds. This allows you to address performance issues before they affect your users.
6. Fixing CORS Errors
CORS (Cross-Origin Resource Sharing) errors can be a headache during development. These errors happen when JavaScript tries to access resources from a different origin, often due to restrictions in the browser.
Check CORS Headers
CORS headers determine which domains can access your API resources. If these headers are missing or incorrectly set, you'll run into trouble. Common error messages include:
- "No 'Access-Control-Allow-Origin' header present"
- "Response to preflight request doesn't pass access control check"
- "Access blocked by CORS policy"
To resolve these issues, review and configure the following headers:
Header | Purpose | Example Value |
---|---|---|
Access-Control-Allow-Origin | Specifies allowed origins | https://yourdomain.com |
Access-Control-Allow-Methods | Defines allowed HTTP methods | GET, POST, PUT, DELETE |
Access-Control-Allow-Headers | Lists permitted custom headers | Content-Type, Authorization |
Access-Control-Allow-Credentials | Enables sending credentials with requests | true |
"Most CORS errors are quick & easy to debug and fix, once you understand the basics." - Tim Perry, Author
Here’s an example to highlight the importance of proper CORS configuration: In October 2023, Coursera faced a problem where students couldn’t access course materials hosted on Amazon S3 due to CORS errors. Their team resolved it by updating the S3 bucket's CORS policy to explicitly include Coursera's domain, restoring access for over 1 million students.
Once headers are correctly set, test your CORS setup across different scenarios.
Test Across Origins
Test how your CORS settings behave in various situations: local development, subdomains, protocol differences, and port variations. Pay close attention to preflight requests and response headers.
For a secure and functional CORS setup:
- Use the
Vary: Origin
header to avoid incorrect caching. - Avoid wildcards (
*
) in production environments. - Handle preflight requests properly for complex requests.
- Match origins exactly when credentials are involved.
Use browser developer tools to inspect request headers and preflight (OPTIONS) responses. This will help you identify and fix configuration issues effectively.
7. Testing Multiple Endpoints
When debugging complex applications, it's often necessary to monitor and test multiple connected endpoints at the same time. After filtering requests and performing performance checks, this step ensures your debugging process covers the entire system. It builds on earlier steps by widening the scope to include multiple endpoints.
Save and Replay Tests
To test multiple endpoints effectively, start by capturing and analyzing HTTP traffic. One useful tool for this is the HTTP Archive (HAR) format, which stores detailed information about requests, including URLs, headers, timings, and status codes.
Testing Phase | Key Actions | What It Helps You Do |
---|---|---|
Capture | Record HTTP traffic in HAR format | Keep a detailed record of requests and responses |
Analysis | Import HAR files into debugging tools | Understand request flows and relationships |
Replay | Modify and resend captured requests | Simulate different scenarios consistently |
Validation | Compare responses across environments | Check for consistent behavior everywhere |
When working with multiple endpoints, pay attention to request dependencies, response timing, header consistency, and the handling of authentication tokens.
Store Test Sessions
Capturing and replaying requests is just the start. Managing sessions can make debugging even more efficient. For instance, tools like the Postman Console can log up to 5,000 messages over 24 hours, giving you a detailed view of your debugging process.
Here are some tips to streamline your workflow:
- Record chains of requests to see how endpoints interact.
- Log key data points for better insights.
- Track configuration changes to identify issues quickly.
- Keep environment settings organized for different scenarios.
To put this into practice:
- Group related endpoints into request collections for better organization.
- Set up environment profiles to test different configurations easily.
- Standardize logging rules across all endpoints.
- Use version tags to manage endpoint versions and ensure compatibility.
These strategies can help you stay organized and make your debugging process smoother.
8. Team Debugging Setup
Setting up shared configurations for HTTP debugging within a team ensures consistency and efficiency. By standardizing these settings, teams can streamline their debugging processes and maintain uniformity across all testing scenarios.
Share Debug Settings
Sharing debugging settings helps teams stay on the same page. Here are some effective methods:
Sharing Method | Best Use Case | Benefits |
---|---|---|
Workspace Sharing | Daily team collaboration | Centralized management, real-time updates |
Shared Rule Lists | Project-specific setups | Easy distribution, version control |
File Export | Offline access | Portable configurations, backup options |
To distribute your debugging rules effectively:
- Create standardized rule sets for common scenarios.
- Keep a detailed record of configuration changes in your team's knowledge base.
- Use version control systems to manage debugging rules.
- Automate rule synchronization across team members to save time.
Multi-Device Setup
Shared configurations are just the beginning. Aligning debugging setups across multiple devices ensures smoother workflows and consistent results.
Setup Phase | Action | Result |
---|---|---|
Initial Configuration | Install certificates, set permissions | Devices can intercept HTTPS traffic |
Environment Sync | Share workspace settings, import rules | Consistent debugging rules |
Validation | Test on all devices, verify responses | Uniform results across devices |
Maintenance | Update rules regularly, verify settings | Continued synchronization |
Here’s how to keep your multi-device setup running smoothly:
- Use a central repository to store and manage debugging configurations.
- Create profiles tailored to specific devices when necessary.
- Schedule regular reviews of configurations to identify and address any issues.
- Document any device-specific limitations to avoid unexpected problems.
Wrapping Up
HTTP debugging can throw a wrench into development, but the right tools and methods can make all the difference. A structured approach improves both workflow and code quality.
Here’s a quick look at how different debugging techniques can streamline development:
Debugging Technique | Benefit to Development | Efficiency Boost |
---|---|---|
Real-time Monitoring | Spot issues instantly | Cuts down on troubleshooting time |
Response Mocking | Speeds up API testing | Removes reliance on backend availability |
Traffic Inspection | Improves visibility | Makes pinpointing problems easier |
Team Collaboration | Standardizes processes | Delivers consistent outcomes |
Modern tools have transformed how developers analyze HTTP traffic. By adopting these methods, teams can identify bugs faster, ensure APIs are more reliable, and maintain high-quality code.
For macOS users, ReqRes offers native debugging features tailored to your setup. Tools like local file mapping, SSL certificate management, and multi-device syncing ensure smooth workflows while tackling common HTTP issues head-on.