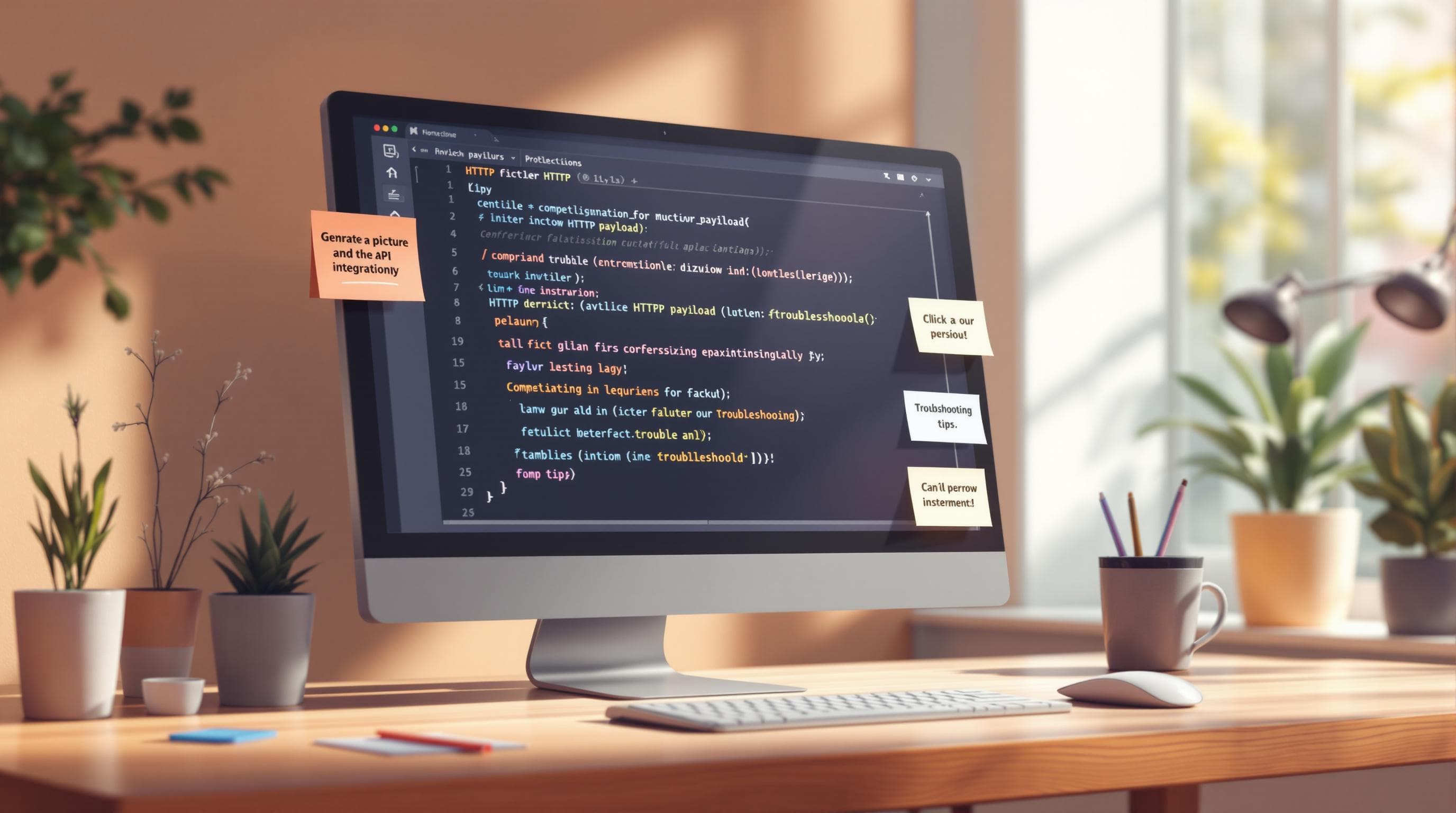
Common Issues in HTTP Payload Debugging
Debugging HTTP payloads can be tricky, but understanding common problems and how to fix them will save you time. Here’s a quick guide to the most frequent issues and their solutions:
- Payload Structure Errors: Missing brackets, wrong data types, or omitted fields in JSON can break your requests.
- Authentication Problems: Invalid tokens, missing API keys, or incorrectly formatted headers block access.
- API Response Issues: Empty responses, incomplete data, or status code errors disrupt functionality.
- Payload Size Challenges: Oversized payloads or compression mismatches can cause transmission problems.
- Rate Limit Errors: Exceeding API limits leads to blocked requests.
How to Fix These Issues:
- Validate JSON structure and compare it to API specs.
- Inspect headers for correct tokens and keys.
- Monitor response data for errors or missing elements.
- Manage rate limits with caching and retry strategies.
Tools like ReqRes simplify debugging by letting you:
- Monitor live HTTP traffic to catch malformed requests.
- Mock API endpoints for testing without a live server.
- Use local files as responses to simulate different scenarios.
Fixing these issues ensures smoother API integrations and faster development. With the right tools and approach, debugging becomes much easier.
Main HTTP Payload Problems
Developers often face three main HTTP payload issues: structure errors, access problems, and response issues. Here's a closer look at each.
Problems with Payload Structure
Some common mistakes in payload structure include:
- Missing brackets or quotes in JSON
- Omitting required fields
- Using the wrong data type (like a string instead of a number)
These errors can cause development delays and trigger system errors. Once the structure is fixed, access issues may still need to be addressed.
Authentication and Authorization Issues
Access problems often stem from authentication or authorization errors, such as:
- Expired or invalid authentication tokens
- Missing or incorrect API keys in the request headers
- Authorization headers formatted incorrectly
Fixing these requires a careful review of request headers and the authentication process. But even after access is granted, response issues can disrupt progress.
Issues with API Responses
Sometimes, the API response itself is the problem. For example:
- Endpoints may return empty response bodies
- Response payloads might contain incomplete data sets
These issues can lead to parsing failures or incomplete functionality.
Payload Size Challenges
Payload size can also cause problems, including:
- Payloads exceeding server size limits
- Data getting truncated due to network restrictions
- Mismatches in compression or encoding settings
Understanding these challenges sets the stage for effective troubleshooting in the next steps.
How to Fix HTTP Payload Issues
After identifying structure, access, and response errors, use these targeted solutions to address the problems.
Check and Fix Payload Format
Ensure your payload structure aligns with API requirements to avoid errors. Start by validating the schema and fixing any mismatches.
Here’s how to validate:
- Check JSON structure and data types: Make sure brackets, quotes, and commas are correctly placed, and verify that values like numbers, strings, and boolean are formatted properly.
- Compare payloads to API specifications: Use schema validation tools to confirm the payload matches the documented API requirements.
Once the format is validated, review both requests and responses to pinpoint any remaining issues.
Track Request and Response Data
Debugging requires clear insight into the data being sent and received.
Steps to monitor effectively:
- Inspect request headers: Focus on the
Content-Type
, authorization tokens, and any custom headers required by the API. - Analyze response data: Look for error status codes, ensure the response body is complete, and check headers that might influence how payloads are processed.
If rate limits are causing issues, tackle them next.
Handle API Rate Limits
Rate limits can disrupt data flow and make debugging harder. Here’s how to manage them:
- Monitor request counts: Ensure you’re staying within the API’s allowed limits.
- Use exponential backoff for retries: Gradually increase retry intervals to avoid overwhelming the API.
- Cache frequent responses: Store commonly used data locally to reduce unnecessary requests.
Fix Authentication Problems
Authentication issues can block payloads from being processed. Solve these problems by:
- Verifying API keys and OAuth tokens: Ensure they’re formatted and sent correctly.
- Refreshing expired tokens: Automate token renewal and log any failures for troubleshooting.
Using ReqRes for HTTP Debugging
ReqRes makes fixing format, access, and response issues easier with its built-in tools. Its native macOS GUI streamlines HTTP payload debugging with three key features:
Monitor Live HTTP Traffic
ReqRes can intercept HTTP(S) requests and responses, displaying them in plain text. This real-time monitoring helps you quickly identify problems like malformed JSON, incorrect headers, or unexpected status codes - without needing extra debug code.
Mock API Endpoints
When the real API isn't available, ReqRes allows you to create mock endpoints with custom responses. This lets you simulate error scenarios, test edge cases, or continue development while waiting for the actual API to go live.
Use Local Files as Responses
The Map Local tool lets you serve content from local files based on specific request rules. With this, you can:
- Test different response payloads without touching the server
- Check how your app handles various response types
- Quickly experiment with and refine payload formats
For example, you can map GET /api/users
to local files like users-success.json
, users-error.json
, or users-empty.json
.
Conclusion: Better Debugging with the Right Tools
Once you've addressed format, authentication, and rate-limit issues, simplify your debugging process with ReqRes. Its real-time HTTP(S) interception and plain-text display make spotting and fixing payload problems much easier. You can mock endpoints, use local files as responses, and keep your development on track even when APIs are down. With its native macOS interface, ReqRes helps teams quickly adapt and stay productive during debugging. Download ReqRes now to streamline your HTTP payload debugging process.
FAQs
How can I tell if there’s an issue with my HTTP payload?
Detecting issues with an HTTP payload often involves noticing unexpected behavior in your application or API. Common signs include:
- Malformed or incomplete data: The payload might be missing fields, have incorrect formats, or include unexpected characters.
- Unusual response codes: Receiving error codes like
400 Bad Request
,500 Internal Server Error
, or other unexpected status codes can indicate a problem. - Slow or failed requests: Delays or timeouts could mean the payload is too large, improperly formatted, or encountering server-side issues.
Using tools like ReqRes can help you intercept and analyze HTTP(S) traffic in real time, making it easier to identify and resolve these issues during development or debugging.
What’s the best way to monitor and debug API requests and responses?
To efficiently monitor and debug your API requests and responses, you can use ReqRes, a native macOS application built for real-time analysis of HTTP(S) traffic. ReqRes lets you intercept and inspect requests and responses in plain text, making debugging quick and straightforward.
This tool works seamlessly as a man-in-the-middle, capturing traffic between your application and SSL web servers without requiring complex setup. Whether you’re testing endpoints, mocking servers, or analyzing payloads, ReqRes simplifies the process for smoother development and troubleshooting.
How can I effectively handle API rate limits during development?
Managing API rate limits is crucial to ensure smooth development and testing without exceeding usage quotas. Here are a few strategies to help:
- Implement Request Throttling: Use a rate-limiting library or custom logic to control how frequently your application makes API calls. This ensures you stay within the allowed limits.
- Monitor API Usage: Keep track of your API request count and reset intervals to avoid hitting the limit unexpectedly. Many APIs provide headers with this information, so be sure to log and analyze it.
- Use Mocking Tools: During development, consider using tools like ReqRes to mock API responses. This allows you to test your application without making real API calls, reducing the risk of hitting rate limits.
By combining these strategies, you can maintain efficient workflows and avoid disruptions caused by rate limit restrictions.